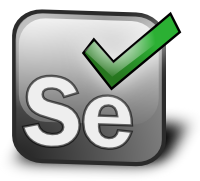
Quick and dirty
After googling quite a bit I couldn’t find a satisfying out-of-the-box solution for cache clearing. I came up with a quick and dirty solution which you can find on stackoverflow.
Simply open a new tab, go to Chrome’s settings, press the right key combo, wait, close the tab and go back. I tested the script in November 2020.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome("path/to/chromedriver.exe")
def delete_cache():
driver.execute_script("window.open('');")
time.sleep(2)
driver.switch_to.window(driver.window_handles[-1])
time.sleep(2)
driver.get('chrome://settings/cleardriverData')
time.sleep(2)
actions = ActionChains(driver)
actions.send_keys(Keys.TAB * 3 + Keys.DOWN * 3) # send right combination
actions.perform()
time.sleep(2)
actions = ActionChains(driver)
actions.send_keys(Keys.TAB * 4 + Keys.ENTER) # confirm
actions.perform()
time.sleep(5) # wait some time to finish
driver.close() # close this tab
driver.switch_to.window(driver.window_handles[0]) # switch back
delete_cache()
|
Just beware that Chromium saves the last user settings. Hence only the first time you should uncheck “Cookies” so your login session doesn’t get deleted.
Simply add a default argument to the function and we are good to go.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
| from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome("path/to/chromedriver.exe")
def delete_cache(first=False):
driver.execute_script("window.open('');")
time.sleep(2)
driver.switch_to.window(driver.window_handles[-1])
time.sleep(2)
driver.get('chrome://settings/cleardriverData')
time.sleep(2)
actions = ActionChains(driver)
actions.send_keys(Keys.TAB * 2) # send right combination
actions.perform()
time.sleep(1)
if first == True:
actions = ActionChains(driver)
actions.send_keys(Keys.DOWN * 4) # send right combination
actions.perform() # theoretically you don't need to add the condition here as the last option is always "everything"
time.sleep(1) # additional "down" clicks won't change anything, anyway let's keep it clean
actions = ActionChains(driver)
actions.send_keys(Keys.TAB * 2) # send right combination
actions.perform()
time.sleep(1)
if first == True: # only first time, chrome saves input from first time
actions = ActionChains(driver)
actions.send_keys(Keys.SPACE) # send right combination
actions.perform()
time.sleep(1)
actions = ActionChains(driver)
actions.send_keys(Keys.TAB * 3 + Keys.ENTER) # confirm
actions.perform()
time.sleep(15) # wait some time to finish
driver.close() # close this tab
driver.switch_to.window(driver.window_handles[0]) # switch back
|